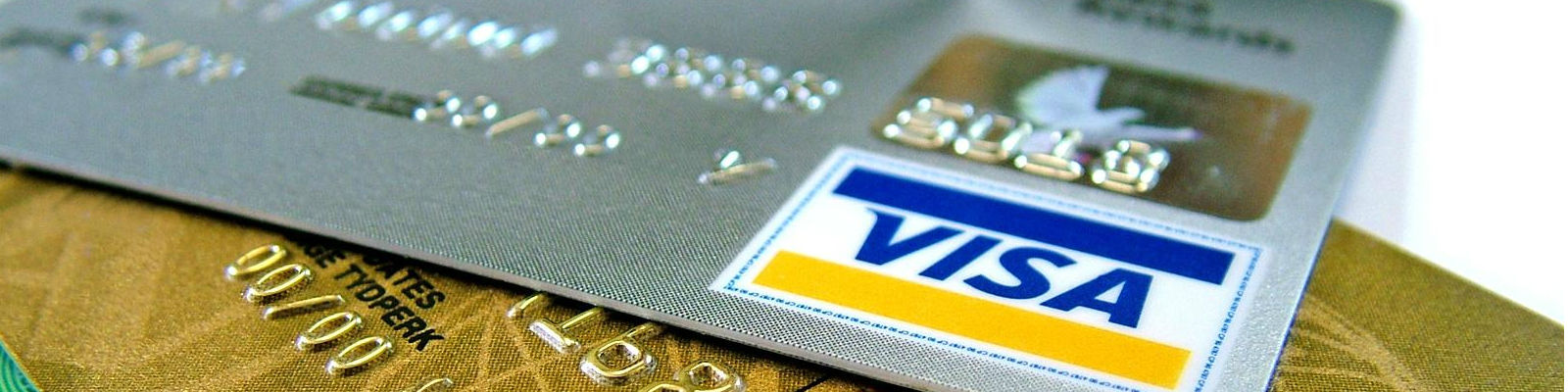
Validate a possible credit card number using C++
To write a program which helps validate a possible credit card number you will need to know two things. Some basic programming skills and the Luhn algorithm.
A short background on the Luhn algorithm
According to online sources the Luhn algorithm or modulus 10 was created by IBM scientist Hans Peter Luhn. The end result of his discovery, patented in 1960, is one that adds a level of integrity to a number which we can use for error reduction. A number known to be generated with this algorithm can be tested for errors after transmitting or recording using this simple method. These types of algorithms act a bit like hidden signatures. An alteration to a number generated with this algorithm is very very likely to change the signature and this change indicates the error. That’s basically it. All credit card numbers are defined with this algorithm built in.
Here it is in action using possible card number 4388576018410707 as an example:
- Beginning from the right, double every second digit (even digits). If doubling results in a two digit number then add the single digits of the two digit number to get a single digit result. In our example: [0x2=0], [0x2=0], [4×2=8], [1×2=2], [6×2=12, 1+2=3], [5×2=10, 1+0=1], [8×2=16, 1+6=7], [4×2=8] These single digit results which I’ve highlighted in bold are then summed [0+0+8+2+3+1+7+8=29]
- Beginning again from right to left add all the of odd digits. In our example: [7+7+1+8+0+7+8+3=41]
- Sum the results from step 1 and step 2. [29+41=70]
If the result from step 3 is divisible by 10 then your number is considered valid!
The following is a simple program which performs this check.
//Author : Daniel Touma (100147753) //Version : 0.1 //Name : Credit Card Number Validator //Description : Checks for a numbers adherence to the Luhn algorithm #include <iostream> #include <string> using namespace std; int main() { string cardNumber; //this will carry the number given by the user. int sum(0), sumOfDoubleEven(0), sumOfOdd(0), evenDigit(0), oddDigit(0); cout << "Welcome to the Credit Card Number Validator." << endl; cout << "This program checks for a numbers adherence to the Luhn algorithm" << endl; //Main loop do { cout << endl << "Please provide a number to validate or type 'n' to quit "; cin >> cardNumber; //gets cardNumber from user if (cardNumber == "n") break; //exits loop on user request //this for loop repeats once for each digit of the cardNumber. //digitPosition decrements by one on each pass from last position to first position for (int digitPosition=cardNumber.length(); digitPosition>0; digitPosition--) { if (digitPosition%2 == 0) { //executes the following code if digitPosition is even oddDigit=(int)cardNumber[digitPosition-1]-'0'; sumOfOdd=sumOfOdd+oddDigit; } else { //executes the following code if digitPosition is odd. evenDigit=((int)cardNumber[digitPosition-1]-'0')*2; evenDigit=evenDigit%10+evenDigit/10; sumOfDoubleEven=sumOfDoubleEven + evenDigit; } } sum=sumOfOdd+sumOfDoubleEven; //sums the result cout << endl <<"The number your provided is "; if (sum%10==0) //executes if sum is divisible by 10 cout << "valid" << endl; else //executes if sum is not divisible by 10 cout << "invalid" << endl; }while (cardNumber != "n"); return 0; }
I have not restricted the size of the number which allows the user to enter a number of almost any size. As you can see the entire program is written inside the main loop, without the use of functions or classes. If you are curious you can see this program rebuilt with functions in an article demonstrating c++ modularity.
Hello,
Just so you know that when you run this code, it defaults to “number you provided is valid/invalid” depending on if the number you type in ends in a zero or not. It doesn’t get passed that. It is helpful to understand with the way you described the steps, but I just figured you would like to know if no one else has mentioned it. Thanks